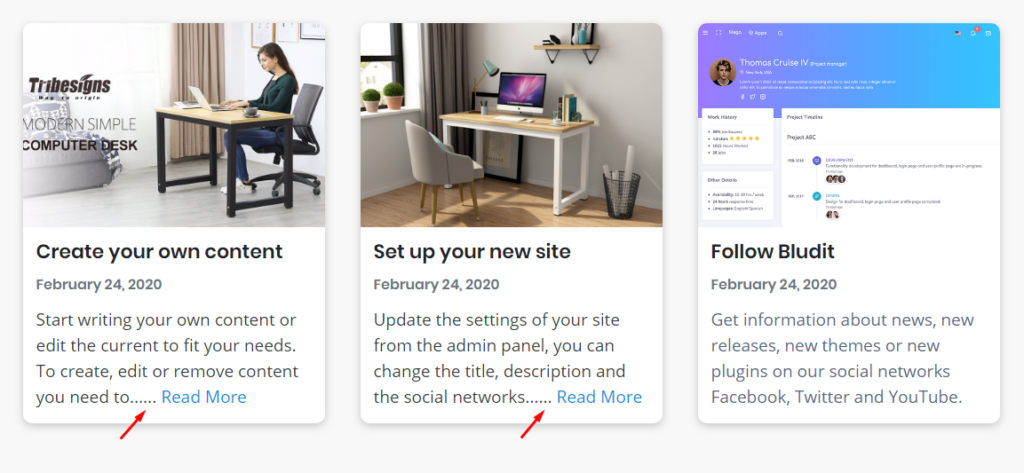
When we have a large paragraph or content and we just have to show few words then what we can do? we need to write some custom code to set limit to our paragraph. And I have a proper example of this code that we can use in this type of situation. In most of my custom blog development I am setting up limit text length in php and that code I am explaining here to achieve this task using few of php default functions like strip_tags(), strlen(), substr(), strrpos()
etc. We can also add read more link at end of this short content after limit is setuped.
To limit text length in php we are using following default php methods :
1. strip_tags() : This function strips a string from HTML, XML, and PHP tags.
parameters : 2 (string required, tags that we want to allowed to run in content)
example :
echo strip_tags("Code try <b><i>catch</i></b>", "<b>");
output : Code try catch
2. strlen() : Returns the length of a string (in bytes) on success, and 0 if the string is empty.
parameters : 1 (string required)
example :
echo strlen("Code try catch");
output : 14
3. substr() : This function returns a specific part of a string.
parameters : 3 (string required, string point in number, length of string in number)
example :
echo substr("Code try catch", 4, 8);
output : try cat
4. strrpos() : The strrpos() function finds the position of the last occurrence of a string inside another string.
parameters : 3 (string required, string to find of last occurrence of string, number from where to start)
example :
echo strrpos("using code try catch blog, we can try to catch result by our code", "catch", 10);
output : 41
Custom function to set limit for content/text :
function limit_text_length($paragraph, $limit, $read_more_link = ""){
// strip tags to avoid breaking any html
$string = strip_tags($paragraph);
if (strlen($string) > $limit) {
// truncate our string
$CutString = substr($string, 0, $limit);
$endPoint = strrpos($CutString, ' ');
// if the string does not contain any space, then it will cut without word basis.
$string = $endPoint? substr($CutString, 0, $endPoint) : substr($CutString, 0);
// add read more link if given
if(!empty($read_more_link)){
$string .= '... <a href="'.$read_more_link.'">Read More</a>';
}
}
return $string;
}
We have developed our custom function by using php default methods. We required 3 parameters which is content, limit in number to break our content at specific number of word and last one is read more link but this last parameter is optional. If we don’t pass this parameter then it will not append read more link.
so in first step we have strip tags from our string or content then we are breaking string by using our limit and then we are finding last space in our new cut string. and then finally we are removing last word after that space because when we use substr then its cutting string by character wise. so we have to display proper word at the end of our cut string.
After this, a final part related to read more link. If we have passed link to our function as a third parameter then this read more link will append or else it will not.
below you can see how to use this function.
Usage of this custom function :
$paragraph = 'Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Auctor neque vitae tempus quam pellentesque nec. In fermentum posuere urna nec. Aliquam ultrices sagittis orci a scelerisque. Eget dolor morbi non arcu risus quis varius. In pellentesque massa placerat duis ultricies lacus sed turpis. Cras ornare arcu dui vivamus arcu. Suspendisse in est ante in nibh mauris cursus. Non odio euismod lacinia at quis risus sed vulputate.';
$limit = 250;
$read_more_link = 'https://codetrycatch.com/';
echo limit_text_length($paragraph, $limit, $read_more_link);
Final output (It will display output like this) :
“Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Auctor neque vitae tempus quam pellentesque nec. In fermentum posuere urna nec. Aliquam ultrices sagittis orci a scelerisque…. Read More”
Conclusion :
As you can see, after this post you can also limit text length using PHP and provide read more link very easily by our custom function. I am using this function in most of my custom blogs or sections development that I want to show in grids like image, title and small description. In future post, we will see some more simple logical tasks that is required for developers in day to day development cycle. So stay connected with my blog to get useful stuff in simple manner, and I will be more happy to get some feedback from you in comment section, and also share this post if its useful for others. Thanks.