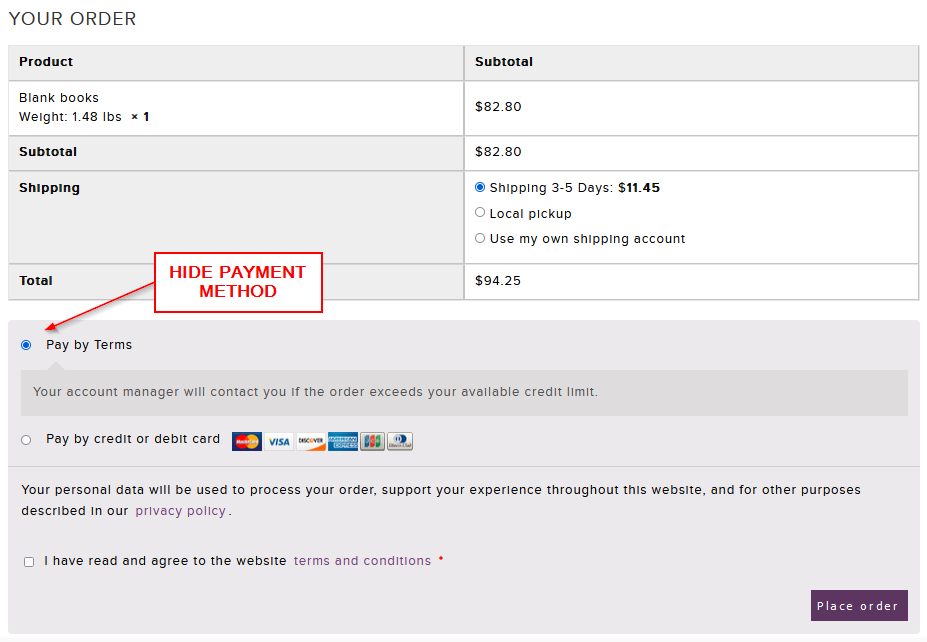
WooCommerce is a powerful platform for building online stores, offering a wide range of payment methods to suit different customer preferences. However, there may be cases where you need to hide certain payment methods from the checkout page for specific reasons. Whether you want to customize the payment options based on user roles, cart contents, or other criteria, WooCommerce provides a straightforward way to achieve this programmatically. In this article, we’ll explore how to programmatically hide payment methods in WooCommerce checkout using the woocommerce_available_payment_gateways filter hook.
The woocommerce_available_payment_gateways filter hook allows you to modify the available payment gateways before they are displayed on the checkout page. By hooking into this filter, you can dynamically alter the list of payment methods based on your custom condition.
Get Payment Method ID from Settings to Hide Payment Methods
Before proceeding with the implementation, you need to find the ID of the payment method you want to hide. To do this, follow these steps:
- Go to your WordPress admin dashboard.
- Navigate to WooCommerce > Settings > Payments.
- Locate the payment method you wish to hide and hover over it to reveal the ID.

Hiding Payment Methods Programmatically
To programmatically hide a payment method, you can use the `woocommerce_available_payment_gateways` filter hook in your theme’s functions.php file or create a custom plugin. Here’s a step-by-step guide on how to do it:
Step 1: Open your functions.php file or create a custom plugin
First, you need to access your theme’s functions.php file or create a custom plugin. Using a custom plugin is recommended to avoid losing your changes during theme updates.
Step 2: Implement the `woocommerce_available_payment_gateways` filter hook
Next, you’ll add the code that utilizes the `woocommerce_available_payment_gateways` filter hook to hide the desired payment method. Here’s an example of the code:
add_filter( 'woocommerce_available_payment_gateways', 'hide_payment_gateway_programmatically' );
function hide_payment_gateway_programmatically( $available_gateways ) {
// Replace 'cheque' with the ID of the payment method you want to hide, in our case we are going to hide cheque payment method
unset( $available_gateways['cheque'] );
return $available_gateways;
}
Make sure to replace “cheque” with the ID of the payment method you want to hide, We are going to hide a cheque payment method in our code snippet.
Step 3: Save and test
After adding the code, save your functions.php file or plugin, and test the checkout page to see if the payment method is hidden based on your specified conditions.
Customization Options
The power of the `woocommerce_available_payment_gateways` filter hook lies in its flexibility. You can customize the payment methods based on various criteria such as:
1. User roles: Show different payment methods for different user roles, like offering exclusive options for premium members.
2. Cart contents: Adjust the available payment methods based on specific products or order amounts.
3. Geographic locations: Display different payment methods based on the customer’s location.
Let us give you some more example to hide payment methods in woocommerce based on different conditions.
Example 1 : Hide Payment Methods for Different User Roles
// Add the following code to your theme's functions.php file or a custom plugin
function hide_payment_gateway_for_user_role($available_gateways) {
// Get the current user role
$current_user = wp_get_current_user();
$user_roles = $current_user->roles;
// Define the user role(s) for which you want to hide the payment gateway
$roles_to_hide_gateway = array('subscriber', 'author', 'editor');
// Define the payment gateway ID you want to hide
$payment_gateway_to_hide = 'cheque';
// Check if the user role is in the roles_to_hide_gateway array and remove the payment gateway if found
if (array_intersect($roles_to_hide_gateway, $user_roles)) {
unset($available_gateways[$payment_gateway_to_hide]);
}
return $available_gateways;
}
add_filter('woocommerce_available_payment_gateways', 'hide_payment_gateway_for_user_role');
In this code snippet, we are hiding cheque payment method for three different user roles as “subscriber, author, editor”. Sof if any user logged in of this user role type then he will not be able to see “cheque”payment method in the checkout page.
Example 2 : Hide Payment Methods if Order Amount is More Than Specific Amount
// Add the following code to your theme's functions.php file or a custom plugin
function hide_payment_gateway_for_order_amount($available_gateways) {
// Define the payment gateway ID you want to hide
$payment_gateway_to_hide = 'cheque';
// Get the cart total amount
$cart_total = WC()->cart->subtotal;
// Define the threshold amount (e.g., $1000)
$threshold_amount = 1000;
// Check if the cart total is more than the threshold amount and remove the payment gateway if it is
if ($cart_total >= $threshold_amount) {
unset($available_gateways[$payment_gateway_to_hide]);
}
return $available_gateways;
}
add_filter('woocommerce_available_payment_gateways', 'hide_payment_gateway_for_order_amount');
We have removed “Cheque” payment method, if order amount is more than or equal to $1000. So user can not see cheque payment method type in checkout page.
Example 3 : Hide Payment Methods if Shipping Country is United States
// Add the following code to your theme's functions.php file or a custom plugin
function hide_payment_gateway_for_country($available_gateways) {
// Define the payment gateway ID you want to hide
$payment_gateway_to_hide = 'cheque';
// Define the country code for which you want to hide the payment gateway
$country_code_to_hide = 'US';
// Get the customer's shipping country
$customer_country = WC()->customer->get_shipping_country();
// Check if the customer's shipping country matches the country code and remove the payment gateway if it does
if ($customer_country === $country_code_to_hide) {
unset($available_gateways[$payment_gateway_to_hide]);
}
return $available_gateways;
}
add_filter('woocommerce_available_payment_gateways', 'hide_payment_gateway_for_country');
So in this code snippet, we have removed cheque payment method for customers who is having “United States (US)” country set in their shipping address.
Conclusion
Programmatically hiding payment methods in WooCommerce checkout provides a seamless and personalized shopping experience for your customers. Utilizing the `woocommerce_available_payment_gateways` filter hook, you can easily modify payment methods on the checkout page to meet your specific business needs.