Some cases we required to create dynamic woocommerce order by programatically. So here we are covering this part below. Woocommerce provided wc_create_order() function to create order by code. This function has array argument and its return wc_order or wc_error.
To the right implementation, in following example we have created one woocommerce product called basic plan. We offering this plan for free. so that means on click of buy we will directly create woocommerce order for that particular user. so for 0 price user dont need to go on cart and checkout page to purchase package. This auto creation of order will skip that cart and checkout process for user.
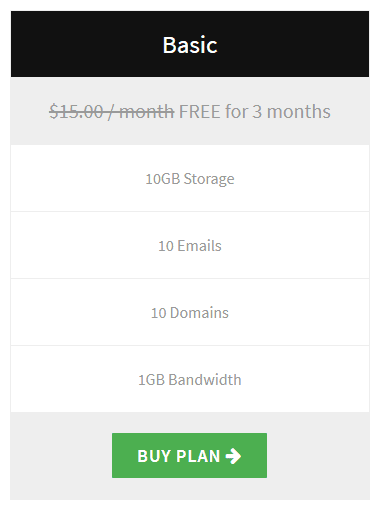
Order in backend
After creation of order dynamically we can see it in woocommerce backend view in orders section.
// shortcode of price block
add_shortcode('free_basic_package', 'free_basic_package_callback');
// callback function of shortcode
function free_basic_package_callback($atts) {
// Get currently logged in user id
$user_id = get_current_user_id();
// declare some variables to show messages of validations
$msg = $success = "";
// check form is submitted or not
if ($_POST['purchase_basic']) {
// check if user logged in then we will go further to create order.
if ($user_id) {
$product_id = $_POST['purchase_basic'];
// set user information by current user id meta.
// here you set your own default address details
$user_info = array(
'first_name' => get_user_meta($user_id, 'billing_first_name', true),
'last_name' => get_user_meta($user_id, 'billing_last_name', true),
'address_1' => get_user_meta($user_id, 'billing_address_1', true),
'address_2' => get_user_meta($user_id, 'billing_address_2', true),
'company' => get_user_meta($user_id, 'billing_company', true),
'email' => get_user_meta($user_id, 'billing_email', true),
'phone' => get_user_meta($user_id, 'billing_phone', true),
'country' => get_user_meta($user_id, 'billing_country', true),
'state' => get_user_meta($user_id, 'billing_state', true),
'postcode' => get_user_meta($user_id, 'billing_postcode', true),
'city' => get_user_meta($user_id, 'billing_city', true),
);
// create the order
$order = wc_create_order();
// The add_product() function has 2 parameters first product and sectiond quantity that we are passing below
$order->add_product(get_product($product_id), 1);
// set user information to set_address function for billing
$order->set_address($user_info, 'billing');
// set user information to set_address function for shipping
$order->set_address($user_info, 'shipping');
// Get payment gateways
$payment_gateways = WC()->payment_gateways->payment_gateways();
// Set payment gateways bacs. you can set any one which is having in $payment_gateways array.
$order->set_payment_method($payment_gateways['bacs']);
// Calculate totals
$order->calculate_totals();
// set custer id to order
$order->set_customer_id($user_id);
// set complated status for this order.
if ($order->update_status('completed', 'Order created dynamically for bronze package', TRUE)) {
// set message if order created successfully and set status as completed
$success = "<h2 style="color: green;">Thanks for join to our basic plan!!</h2>";
}
} else {
// set message if user not logged in to site
$msg = "<h2 style="color: red;">Please login first to buy package!!</h2>";
}
}
// return html form by shortcode callback funciton
$form = '<style>*{box-sizing:border-box}.columns{float:left;width:33.3%;padding:8px}.price{list-style-type:none;border:1px solid #eee;margin:0;padding:0;-webkit-transition:.3s;transition:.3s;list-style:none!important;padding-left:0px!important}.price:hover{box-shadow:0 8px 12px 0 rgba(0,0,0,.2)}.price .header{background-color:#111;color:#fff;font-size:25px}.price li{border-bottom:1px solid #eee;padding:20px;text-align:center}.price .grey{background-color:#eee;font-size:20px}.button{background-color:#4caf50;border:none;color:#fff;padding:10px 25px;text-align:center;text-decoration:none;font-size:18px}@media only screen and (max-width:600px){.columns{width:100%}}</style>
' . $success . '
' . $msg . '
<form action="" class="basic_form" method="post" style="text-align: center;">
<div class="columns">
<ul class="price">
<li class="header">Basic</li>
<li class="grey"><span style="text-decoration: line-through;">$15.00 / month</span> FREE for 3 months</li>
<li>10GB Storage</li>
<li>10 Emails</li>
<li>10 Domains</li>
<li>1GB Bandwidth</li>
<li class="grey">
<button class="btn btn-theme button" type="submit">Buy Plan
<i aria-hidden="true" class="fa fa-arrow-right"></i>
</button>
<input name="purchase_basic" type="hidden" value="' . $atts['productid'] . '">
</li>
</ul>
</div>
</form>';
return $form;
}<span style="font-family: arial; font-size: large;">
</span>